In this post I am taking care of some basic staff like draw rectangular, line, circle, image etc in HTML5 CANVAS. These are the basic structure but we need to use each of them in advanced functions.
Almost every CANVAS JavaScript functions have 2 common lines, one which we take the CANVAS in a veriable and then get the 2d context of the variable
So our first part of code alaways same, like
Like, previous post we should place the JS code in the bottom of the page.
Var canvas = document.getElementById(‘canvas’);
Var getcontext = canvas.gerContext(‘2d’);
//We will only discuss the 2d function in this series of HTML5 CANVAS posts.
And into the body section, put this code like mention above.
<canvas width=”500” height=”300” id=”canvas”></canvas>
Lets start draw something.
getcontext.fillStyle=”#000”;
//adding the background color to the context which we will assign it to the rect function
getcontext.fillRect (10,10,480,280);
//We can also try strokeRect method for a rectangular stroke. The first 2 parameters are coordinates, I am assigning it to x as 10 and y as 10 then the width and Height as 480, 280.
Stroke() method create he CANVAS line.
getcontext.strokeStyle="#ff0000";
getcontext.moveTo(0,0);
getcontext.lineTo(500,300);
getcontext.stroke();
getcontext.beginPath(); // Begin the path
getcontext.arc(120,120,70,0,2*Math.PI); // arc method help to create circle
getcontext.fillStyle="#8ED6FF";
getcontext.strokeStyle= "#ff0000";
getcontext.fill();
getcontext.stroke();
Here we used both stoke and fill, so we need to use both the function .fill and .stroke
Same is other we have the fillStyle and stokeStyle for text print.
getcontext.fillStyle="#ff0000";
getcontext.strokeStyle="#000";
getcontext.font = "30px Arial"; //Assigning font size and font family
getcontext.fillText("Hello World",170,140); // Fill the text with color red k
getcontext.strokeText("Hello World",175,140); // Stroke the text with black
var imageObj = new Image();
imageObj.onload = function() {
context.drawImage(imageObj, 150, 50);
};
imageObj.src = 'https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEg_UZDHPD5jmf_4vTk8dqAsGkcwIzQt3tnWOv-iDeql30H8Zyevb87Bs7yCEG8w7JXElblErqxsqylZRaZ0ETyM-xKxARxRpoiuq2seso-7-FtA5cR29uLxZyHFFJCsYKfs8ZRn-FFkeaY/s1600/fabicon-big.jpg';
We will create gradient and will apply to rectangle, circle.
grd.addColorStop(0,'#ff0000'); // Add color based on your requirement
grd.addColorStop(.4,'#000');
grd.addColorStop(1,'#0000ff');
getcontext.fillStyle= grd; // Applying the gradient.
getcontext.fillRect(150,50,200,200);
grd.addColorStop(0, 'red'); // Add color based on your requirement
grd.addColorStop(1, '#fff');
getcontext.fillStyle= grd; // Applying gradient
getcontext.fillRect(10, 10, 200,200);
These are the very basic diagram for HTML5 CANVAS. In the next post I will go through some more advanced diagram and it features.
Thanks for visiting.Please support us by shearing our posts and like your social pages.
Please subscribe for our future posts.
start the code:
Like the previous post, for creating a HTML5 CANVAS page, we placed a CANVAS tag with width 500, height 300 into the body section and JavaScript functions into the bottom of the HTML before the body tag close(in the previous post we discuss the possibilities for placing JavaScript codes into the page).Almost every CANVAS JavaScript functions have 2 common lines, one which we take the CANVAS in a veriable and then get the 2d context of the variable
So our first part of code alaways same, like
Like, previous post we should place the JS code in the bottom of the page.
Var canvas = document.getElementById(‘canvas’);
Var getcontext = canvas.gerContext(‘2d’);
//We will only discuss the 2d function in this series of HTML5 CANVAS posts.
And into the body section, put this code like mention above.
<canvas width=”500” height=”300” id=”canvas”></canvas>
Lets start draw something.
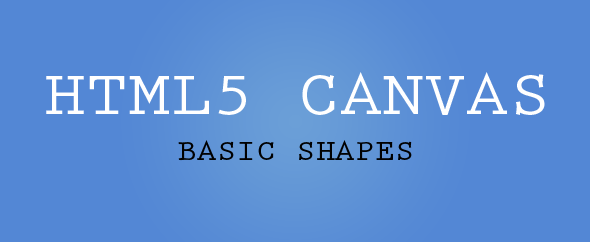
1. Rect :
For drawing a rectangular we need add the style our context variable first and then either stroke or fill the rectangular. Like I am just filling the background:getcontext.fillStyle=”#000”;
//adding the background color to the context which we will assign it to the rect function
getcontext.fillRect (10,10,480,280);
//We can also try strokeRect method for a rectangular stroke. The first 2 parameters are coordinates, I am assigning it to x as 10 and y as 10 then the width and Height as 480, 280.
OUTPUT
Line
For creating a line into CANVAS moveTo is the function start the line coordinate, here I use 0,0 and the lineTo method end the stroke.These function just start the point and move it to the end.Stroke() method create he CANVAS line.
getcontext.strokeStyle="#ff0000";
getcontext.moveTo(0,0);
getcontext.lineTo(500,300);
getcontext.stroke();
OUTPUT
Circle
For creating a circle in CANVAS beginPath() is for the begin the path for circle. The arc method help you create a circle or sphere.getcontext.beginPath(); // Begin the path
getcontext.arc(120,120,70,0,2*Math.PI); // arc method help to create circle
getcontext.fillStyle="#8ED6FF";
getcontext.strokeStyle= "#ff0000";
getcontext.fill();
getcontext.stroke();
Here we used both stoke and fill, so we need to use both the function .fill and .stroke
OUTPUT
Sphere
We just need to add Scaleto circle for make it sphere.Text
In the last post where we have crated the very first CANVAS function “Hello World”, we used this text method. Now here is the description how it work.Same is other we have the fillStyle and stokeStyle for text print.
getcontext.fillStyle="#ff0000";
getcontext.strokeStyle="#000";
getcontext.font = "30px Arial"; //Assigning font size and font family
getcontext.fillText("Hello World",170,140); // Fill the text with color red k
getcontext.strokeText("Hello World",175,140); // Stroke the text with black
OUTPUT
Image
For the image it could be form the other website or could be local file but we need to run our function after the image load.var imageObj = new Image();
imageObj.onload = function() {
context.drawImage(imageObj, 150, 50);
};
imageObj.src = 'https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEg_UZDHPD5jmf_4vTk8dqAsGkcwIzQt3tnWOv-iDeql30H8Zyevb87Bs7yCEG8w7JXElblErqxsqylZRaZ0ETyM-xKxARxRpoiuq2seso-7-FtA5cR29uLxZyHFFJCsYKfs8ZRn-FFkeaY/s1600/fabicon-big.jpg';
OUTPUT
Gradient
We have two types of gradients.- createLinearGradient(x,y,x1,y1) - creates a linear gradient.
- createRadialGradient(x,y,r,x1,y1,r1) - creates a radial/circular gradient.
We will create gradient and will apply to rectangle, circle.
Linear Gradient:
var grd = getcontext.createLinearGradient(0,0,500,0);grd.addColorStop(0,'#ff0000'); // Add color based on your requirement
grd.addColorStop(.4,'#000');
grd.addColorStop(1,'#0000ff');
getcontext.fillStyle= grd; // Applying the gradient.
getcontext.fillRect(150,50,200,200);
OUTPUT
Radial Gradient:
var grd = getcontext.createRadialGradient(100,120,2,100,120,60); // Create the radial gradientgrd.addColorStop(0, 'red'); // Add color based on your requirement
grd.addColorStop(1, '#fff');
getcontext.fillStyle= grd; // Applying gradient
getcontext.fillRect(10, 10, 200,200);
OUTPUT
These are the very basic diagram for HTML5 CANVAS. In the next post I will go through some more advanced diagram and it features.
Thanks for visiting.Please support us by shearing our posts and like your social pages.
Please subscribe for our future posts.
No comments:
Post a Comment